Let us make a WP theme for the site simple.miliczki.sk using the Bootstrap css framework. The basic theme is made based on hostiger.com “How to Create a WordPress Theme: 5-Step Process with Code”. The only difference is that instead of CSS normalization using
<?php // This function enqueues the Normalize.css for use. The first parameter is a name for the stylesheet, the second is the URL. Here we // use an online version of the css file. function add_normalize_CSS() { wp_enqueue_style( 'normalize-styles', "https://cdnjs.cloudflare.com/ajax/libs/normalize/7.0.0/normalize.min.css"); } add_action('wp_enqueue_scripts', 'add_normalize_CSS');
we rely on Bootsrap’s Reboot, collection of element-specific CSS changes to provide an elegant, consistent, and simple baseline to build upon (normalizing Page defaults, fonts, …). This is included in header.php
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
The first page with original normalize.css:
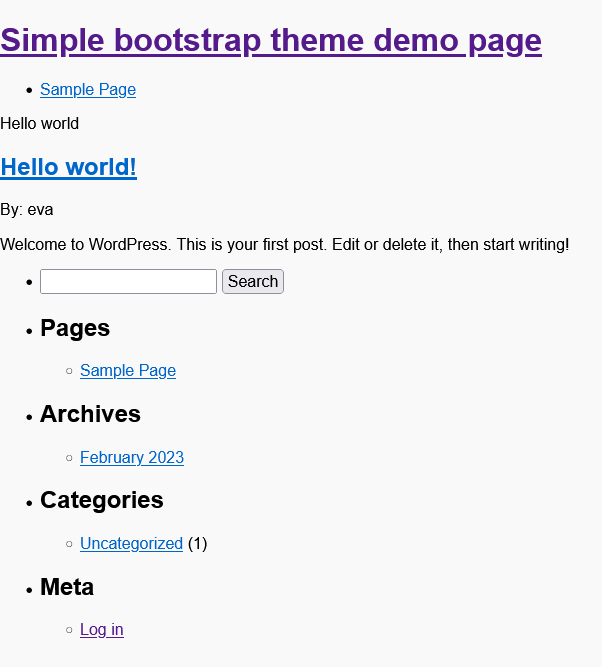
And with Reboot:
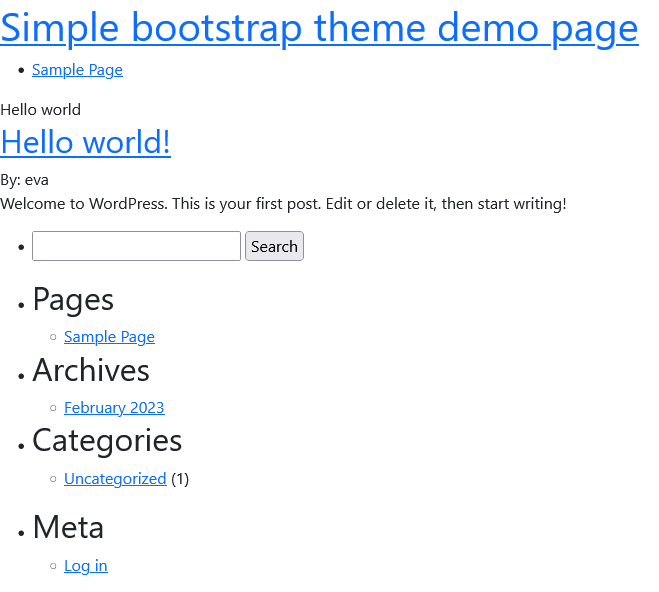
How does the theme work?
The main theme file is index.php. It starts with
<?php get_header(); ?>
header.php
Here header.php is called. Here in header.php, in the <head> and before it, we set the title, metadata, load Bootstrap’s stylesheet:
<!DOCTYPE html> <html <?php language_attributes(); ?> <head> <title><?php bloginfo('name'); ?> » <?php is_front_page() ? bloginfo('description') : wp_title(''); ?></title> <meta charset="<?php bloginfo( 'charset' ); ?>"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>"> <!-- Bootstrap CSS --> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous"> <?php wp_head(); ?> </head>
The part of the result of this is this html code (then there is a lot more of code generated by <?php wp_head(); ?>) :
<!DOCTYPE html> <html lang="en-US" <head> <title>Simple bootstrap theme demo page » Demo page for somple bootstrap theme</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://simple.miliczki.sk/wp-content/themes/simplebootstrap/style.css">
The <header> is generated by this section in header.php
<header> <h1><a href="<?php echo esc_url(home_url( '/' )); ?>"><?php bloginfo('name'); ?></a></h1> </header>
After creating a simple menu through WP admin, we have got a menu generated by
<?php wp_nav_menu( array( 'header-menu' => 'header-menu' ) ); ?>
Here we can see the Header and the Menu:
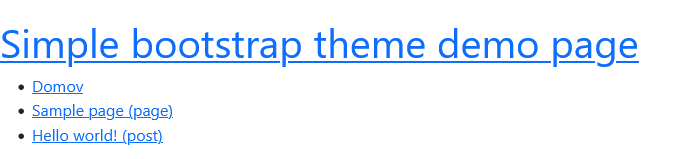
Here header.php ends.
<main>
Next, in index.php, the <main> section of the <body> is defined. Here, we start a <section> (In HTML, a section is a semantic element for creating standalone sections in a web page. These sections should be made up of related content, like contact information.The section element should only be used if there isn’t a more specific element to represent the related content. blog.hubspot.com) for articles. In the section, we cycle through available posts and display them with this cycle.
<?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?> <article> <header> <h2><a href="<?php the_permalink(); ?>" title="<?php the_title_attribute(); ?>"><?php the_title(); ?></a></h2> By: <?php the_author(); ?> </header> <?php the_excerpt(); ?> </article> <?php endwhile; else : ?>
An <article> is should be used to represent a unit of content on a web page that could, in principle, exist on its own or be reused. Both <section> and <article> This helps search engines, browsers, assistive technologies, and other developers understand the different parts of a web page (more details e.g. on blog.hubspot.com). And this is the result for the sample posts:
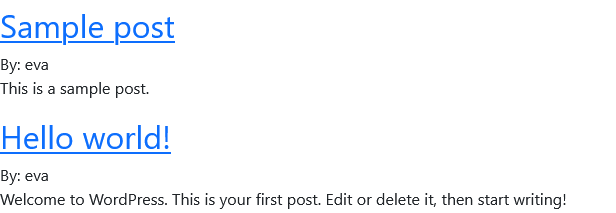
Her we have used “The Loop” which is PHP code used by WordPress to display posts.
The last call in main, after </section> is for the sidebar <?php get_sidebar(); ?> which is in the sidebar.php file in our case.
sidebar.php
The rest of the page is not yet clear to me. It seems that a default sidebar is displayed at the bottom of the page
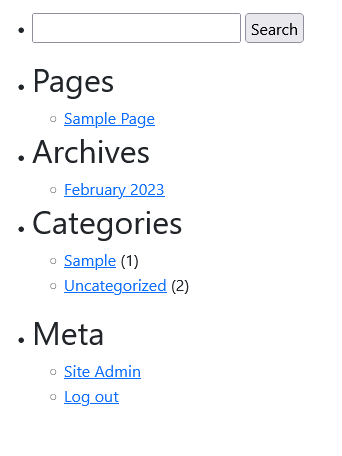
But I am not sure where it is defined.
List of files
index.php
<?php get_header(); ?> <main> <section> <?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?> <article> <header> <h2><a href="<?php the_permalink(); ?>" title="<?php the_title_attribute(); ?>"><?php the_title(); ?></a></h2> By: <?php the_author(); ?> </header> <?php the_excerpt(); ?> </article> <?php endwhile; else : ?> <article> <p>Sorry, no posts were found!</p> </article> <?php endif; ?> </section> <?php get_sidebar(); ?> </main> <?php get_footer(); ?>
header.php
<!DOCTYPE html> <html <?php language_attributes(); ?> <head> <title><?php bloginfo('name'); ?> » <?php is_front_page() ? bloginfo('description') : wp_title(''); ?></title> <meta charset="<?php bloginfo( 'charset' ); ?>"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>"> <!-- Bootstrap CSS --> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous"> <?php wp_head(); ?> </head> <body <?php body_class(); ?>> <!-- Option 2: Separate Popper and Bootstrap JS --> <!-- https://getbootstrap.com/docs/5.1/getting-started/introduction/#starter-template --> <script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.10.2/dist/umd/popper.min.js" integrity="sha384-7+zCNj/IqJ95wo16oMtfsKbZ9ccEh31eOz1HGyDuCQ6wgnyJNSYdrPa03rtR1zdB" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.min.js" integrity="sha384-QJHtvGhmr9XOIpI6YVutG+2QOK9T+ZnN4kzFN1RtK3zEFEIsxhlmWl5/YESvpZ13" crossorigin="anonymous"></script> <header> <h1><a href="<?php echo esc_url(home_url( '/' )); ?>"><?php bloginfo('name'); ?></a></h1> </header> <?php wp_nav_menu( array( 'header-menu' => 'header-menu' ) ); ?>
footer.php
</body> </html>
sidebar.php
<?php if ( is_active_sidebar( 'sidebar' ) ) : ?> <aside id="primary-sidebar" class="primary-sidebar widget-area" role="complementary"> <?php dynamic_sidebar( 'sidebar' ); ?> </aside> <?php endif; ?>
functions.php
// Register a new sidebar simply named 'sidebar' function add_widget_support() { register_sidebar( array( 'name' => 'Sidebar', 'id' => 'sidebar', 'before_widget' => '<div>', 'after_widget' => '</div>', 'before_title' => '<h2>', 'after_title' => '</h2>', ) ); } // Hook the widget initiation and run our function add_action( 'widgets_init', 'add_widget_support' ); // Register a new navigation menu function add_Main_Nav() { register_nav_menu('header-menu',__( 'Header Menu' )); } // Hook to the init action hook, run our navigation menu function add_action( 'init', 'add_Main_Nav' ); ?>