I have tried several options of how to integrate Bootsrap with WordPress. According to Bootstrap guidelines, we need Bootstrap’s css to be loaded before all the other css files.
The scripts for Bootstrap’s JavaScript should be loaded before the closing </body>
tag.
The source files can be either downloaded on the server, or accessed via CDN links.
If we want to have the possibility to edit Bootstrap styles, then we have to download source Bootstrap files. However, these files (.scss) have to be compiled to .css to be used in WordPress. For this purpose I use the Happy WP SCSS Compiler plugin. So this is the way I have inserted Bootstrap into this WordPress site.
1. Install Bootstrap in WordPress using Bootstrap source files
This is done in the following steps:
1.1 Child theme
First, I have made a child theme, called blocksy-child
, the steps were described in this blog post.
1.2 Bootstrap source files
I have donwloaded the Bootstrap source files from here. I have dowloaded ONLY the source files, not Sass compiler, nor Autoprefixer.
My file structure is the following:
blocksy-child/ |--scss |--bootstrap.scss |--css |--bootstrap |--js |--scss
1.3 Scss file for Bootstrap
My bootstrap.scss file looks like this – I have included all of Bootstrap.
@import url("https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.3/font/bootstrap-icons.css"); $primary: #ff6d00; $secondary: #cfa9ef; $danger: #5a189a; $success: #4D962B; $warning: orange; $info: aqua; $body-bg: #f3f3f0; @import "../bootstrap/scss/bootstrap";
1.4 Compiling scss to css in WordPress
I have installed the Happy WP SCSS Compiler plugin. The SCSS Folder Path is set to /scss/ and CSS Folder Path is set to /css/. The Auto Enqueue CSS
files is set to NO. The source scss file is compiled to css file every time it has changed.
1.5 Including compiled .css file in WordPress
This was the trickiest part for me. I had two major problems:
a) Bootstrap style file should be loaded as FIRST
The tackling of this problem is described later in this article.
b) The newest version of the css file has to be loaded.
For this purpose, I have used the filemtime()
php function, as described here.
My functions.php
file is this:
<?php //Child theme add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles',1 ); function my_theme_enqueue_styles() { $parenthandle = 'blocksy-style'; // This is 'twentyfifteen-style' for the Twenty Fifteen theme. $theme = wp_get_theme(); wp_enqueue_style( $parenthandle, get_template_directory_uri() . '/style.css', array(), // If the parent theme code has a dependency, copy it to here. $theme->parent()->get( 'Version' ) ); wp_enqueue_style( 'child-style', get_stylesheet_uri(), array( $parenthandle ), $theme->get( 'Version' ) ); } //Bootstrap function bootstrap_style() { wp_register_style('bootstrap5', get_theme_root_uri().'/blocksy-child/css/style.css', $deps = array(), $ver = filemtime( get_stylesheet_directory().'/css/style.css'), $media = 'all'); array_unshift(wp_styles()->queue, 'bootstrap5'); wp_enqueue_style('bootstrap5'); } add_action( 'wp_enqueue_scripts', 'bootstrap_style'); ?>
2. Using CDN links
Here the last method is correct and working.
2.1 Inserting links to Bootstrap files into header.php and footer.php of the current theme – not correct
Inserting links to Bootstrap files into header.php and footer.php of the current theme, as described in this post, works. But is not recommended as each theme update deletes the files. The result is fine, as we expected:
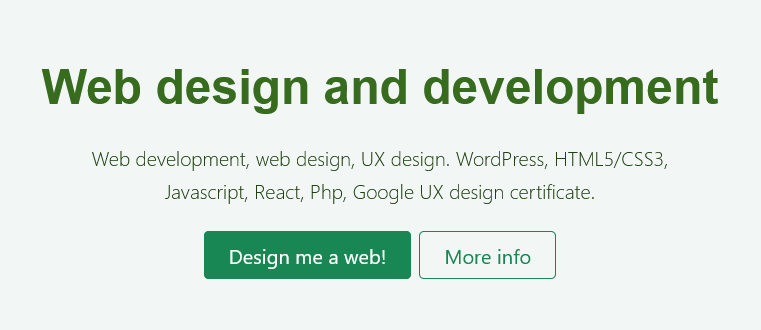
2.2 Using a plugin to insert links to Bootstrap – not perfect
Using Code snippets WP plugin to inserts links to Bootstrap’s css and scripts. This is a problem, since Bootstrap’s css file is not loaded as first, in fact this stylesheet is loaded as the last one. Therefore, the Bootstrap’s Reboot normalization rewrites my own settings. The result is white background of the page and black heading.
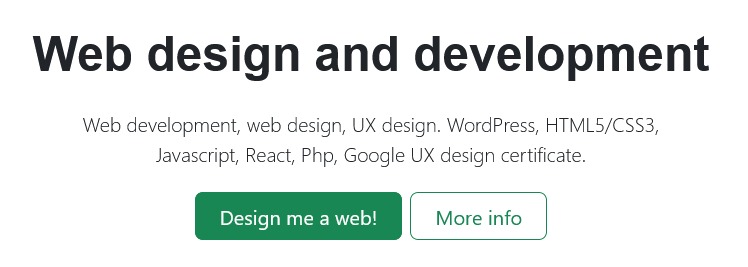
2.3 Using a child theme to insert links Bootstrap using only wp_enqueue_style – not perfect
Making of a child theme was described in this blog post. The proper way to insert links to additional css and js files is using e.g. wp_enqueue_style(), wp_enqueue_script()
and then adding it to a hook using add_action()
. Usualy wp_enqueue_scripts
hook is used . Inspiration on wordpress.stackexchange. From the file functions.php:
function my_scripts() { wp_enqueue_style('bootstrap4', 'https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css'); wp_enqueue_script( 'boot1','https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js', array( 'jquery' ),'',true ); wp_enqueue_script( 'boot2','https://cdn.jsdelivr.net/npm/@popperjs/core@2.11.6/dist/umd/popper.min.js', array( 'jquery' ),'',true ); wp_enqueue_script( 'boot3','https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.min.js', array( 'jquery' ),'',true ); } add_action( 'wp_enqueue_scripts', 'my_scripts' );
Still, the Bootstrap’s css is not first, so we have the same problem as in 2.
2.4 Inserting links using wp_register_script() and array_unshift() – correct and working
This solution, from wordpress.stackexchange.com is working. Bootsrap’s css is now loaded as the first one.
function bootstrap_style() { wp_register_style('bootstrap5', 'https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css'); array_unshift(wp_styles()->queue, 'bootstrap5'); wp_enqueue_style('bootstrap5'); } add_action( 'wp_enqueue_scripts', 'bootstrap_style');
To put Bootstrap JS files just before the closing body tag, we use the wp_footer
hook (instructions from wordpress.org/support/):
function bootstrap_scripts() { wp_enqueue_script( 'boot1','https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js', array( 'jquery' ),'',true ); wp_enqueue_script( 'boot2','https://cdn.jsdelivr.net/npm/@popperjs/core@2.11.6/dist/umd/popper.min.js', array( 'jquery' ),'',true ); wp_enqueue_script( 'boot3','https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.min.js', array( 'jquery' ),'',true ); } add_action( 'wp_footer', 'bootstrap_scripts');
Now all works as it should…as you can see on this webpage.